Soru

Part 2 (30pts) Write a Python function removeDuplicates() that takes a list as parameter and returns a new list in which duplicate items are removed from the list. Hint: Think about appending the items of the list to an empty list and prevent appending an existing item. Write Python statements that creates a list that has duplicate elements, call the above function to get a list of unique values and print this new list. Part 2: The original lis t is:
Çözüm
4.6290 Voting
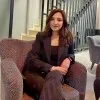
Ümran
Usta · 5 yıl öğretmeniUzman doğrulaması
Cevap
Here's a Python function that removes duplicates from a list:<br />```python<br />def removeDuplicates(lst):<br /> unique_lst = []<br /> for item in lst:<br /> if item not in unique_lst:<br /> unique_lst.append(item)<br /> return unique_lst<br />```<br />This function takes a list as a parameter and returns a new list with unique values. It uses an empty list `unique_lst` to store the unique values, and a for loop to iterate through each item in the input list. The `if` statement checks if the current item is already in the `unique_lst`. If it's not, the item is appended to the `unique_lst`.<br /><br />Here's an example of how to use this function:<br />```python<br /># create a list with duplicate elements<br />original_lst = [1, 2, 3, 2, 4, 5, 6, 7, 1, 4]<br /><br /># call the removeDuplicates function to remove duplicates<br />new_lst = removeDuplicates(original_lst)<br /><br /># print the new list<br />print(new_lst)<br />```<br />This will output the following list with unique values:<br />```<br />[1, 2, 3, 4, 5, 6, 7]<br />```<br />Note that the order of the elements may not be preserved, as the `removeDuplicates` function does not guarantee the order of the unique values in the output list.
Derecelendirmek için tıklayın: