Soru

C Programming Assignment: Student Marks Analysis Objective: Write a C program that performs the following tasks related to student marks analysis: 1. Input: Read the number of students (n) Read the marks of n students into an array using a function. 2. Processing: Use a function to calculate the average marks Use another function to find the highest mark and the lowest mark. Use a function to count how many students scored above average. 3. Output: Print all the marks , the average mark, the highest mark, the lowest mark, and the number of students scoring above average.
Çözüm
4.4255 Voting
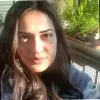
Jihan
Profesyonel · 6 yıl öğretmeniUzman doğrulaması
Cevap
Here is a sample C program that performs the tasks mentioned in the objective:<br /><br />```c<br />#include <stdio.h><br /><br />// Function to read the marks of n students into an array<br />void readMarks(int marks[], int n) {<br /> for (int i = 0; i < n; i++) {<br /> printf("Enter mark for student %d: ", i + 1);<br /> scanf("%d", &marks[i]);<br /> }<br />}<br /><br />// Function to calculate the average marks<br />float calculateAverage(int marks[], int n) {<br /> float sum = 0;<br /> for (int i = 0; i < n; i++) {<br /> sum += marks[i];<br /> }<br /> return sum / n;<br />}<br /><br />// Function to find the highest mark and the lowest mark<br />void findHighestLowest(int marks[], int n, float *highest, float *lowest) {<br /> *highest = marks[0];<br /> *lowest = marks[0];<br /> for (int i = 1; i < n; i++) {<br /> if (marks[i] > *highest) {<br /> *highest = marks[i];<br /> }<br /> if (marks[i] < *lowest) {<br /> *lowest = marks[i];<br /> }<br /> }<br />}<br /><br />// Function to count how many students scored above average<br />int countAboveAverage(int marks[], int n, float avg) {<br /> int count = 0;<br /> for (int i = 0; i < n; i++) {<br /> if (marks[i] > avg) {<br /> count++;<br /> }<br /> }<br /> return count;<br />}<br /><br />int main() {<br /> int n;<br /> printf("Enter the number of students: ");<br /> scanf("%d", &n);<br /><br /> int marks[n];<br /> readMarks(marks, n);<br /><br /> float avg = calculateAverage(marks, n);<br /> float highest, lowest;<br /> findHighestLowest(marks, n, &highest, &lowest);<br /><br /> int aboveAvg = countAboveAverage(marks, n, avg);<br /><br /> printf("Marks: ");<br /> for (int i = 0; i < n; i++) {<br /> printf("%d ", marks[i]);<br /> }<br /> printf("\nAverage mark: %.2f\n", avg);<br /> printf("Highest mark: %.2f\n", highest);<br /> printf("Lowest mark: %.2f\n", lowest);<br /> printf("Number of students scoring above average: %d\n", aboveAvg);<br /><br /> return 0;<br />}<br />```<br /><br />This program will prompt the user to enter the number of students and their respective marks. It will then calculate the average, highest, and lowest marks, and count the number of students who scored above the average. Finally, it will print all the marks, the average mark, the highest mark, the lowest mark, and the number of students scoring above average.
Derecelendirmek için tıklayın: