Soru

Your Task: Python While Nested For Loop 1. Write a Python program that utilizes a while nested for loop to generate a number pyramid. The program should perform the following actions: Requirements: Use a loop structure to generate the pyramid pattern. Each line should start with the number 1 and incrementally increase up to the line number. Avoid hardcoding the output;your code should riynamically generate each line. Ensure that the program gracefully handles user inputs, and after the loop exits, print a concluding message. Sample Output:
Çözüm
3.2243 Voting
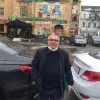
Cemil
Elit · 8 yıl öğretmeniUzman doğrulaması
Cevap
Here is a Python program that utilizes a while nested for loop to generate a number pyramid:<br /><br />```python<br />def number_pyramid(n):<br /> count = 1<br /> while count <= n:<br /> for i in range(1, count + 1):<br /> print(i, end=" ")<br /> print() # Newline after each line of the pyramid<br /> count += 1<br /><br /># Get user input<br />n = int(input("Enter the number of lines for the pyramid: "))<br /><br /># Generate the pyramid<br />number_pyramid(n)<br /><br /># Print a concluding message<br />print("You have generated a number pyramid with", n, "lines.")<br />```<br /><br />### Explanation:<br />1. **Function Definition (`number_pyramid`)**:<br /> - The function `number_pyramid` takes an integer `n` as input, which represents the number of lines in the pyramid.<br /> - A `while` loop is used to iterate as long as `count` is less than or equal to `n`.<br /><br />2. **Inner `for` Loop**:<br /> - The `for` loop iterates from 1 to `count`, printing each number followed by a space.<br /> - After each iteration of the `for` loop, a newline is printed to move to the next line of the pyramid.<br /><br />3. **Incrementing `count`**:<br /> - After each iteration of the `while` loop, `count` is incremented by 1.<br /><br />4. **User Input**:<br /> - The program prompts the user to enter the number of lines for the pyramid.<br /> - The input is converted to an integer and passed to the `number_pyramid` function.<br /><br />5. **Concluding Message**:<br /> - After the `while` loop exits, a concluding message is printed indicating the number of lines generated.<br /><br />This program will dynamically generate a number pyramid based on the user's input.
Derecelendirmek için tıklayın: