Soru

A: 3 T: 3 C: 3 G: 3 Date: 16/10/2024 due on 30/10/2024 Problem: DNA Sequence Analysis You are given a DNA sequence, which is a string composed of the characters 'A', 'T', 'C' and 'G' Write a program that counts the occurrences of each nucleotide ( 'A' , "T", "C", and 'G' ) in the sequence. Solve this problem using Java implementing it in three different paradigms: 1. Imperative Approach: Write a loop-based solution where you iterate over the sequence, count each nucleotide's occurrences, and display the result. 2. Object-Oriented Approach:Create a class DNAAnalyser that holds the DNA sequence and provides a method countNucleotides to count and return the occurrences of each nucleotide. 3. Functional Approach: Use Java's functional programming features (e.g., Streams and Collectors.groupingBy) to count the occurrences of each nucleotide in a functional style. Input:"ATCGATCGATCG"
Çözüm
3.5202 Voting
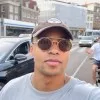
Veli
Usta · 5 yıl öğretmeniUzman doğrulaması
Cevap
1. Imperative Approach:<br />```java<br />public class DNASequenceAnalysis {<br /> public static void main(String[] args) {<br /> String sequence = "ATCGATCGATCG";<br /> int[] count = new int[4];<br /><br /> for (int i = 0; i < sequence.length(); i++) {<br /> char nucleotide = sequence.charAt(i);<br /> switch (nucleotide) {<br /> case 'A':<br /> count[0]++;<br /> break;<br /> case 'T':<br /> count[1]++;<br /> break;<br /> case 'C':<br /> count[2]++;<br /> break;<br /> case 'G':<br /> count[3]++;<br /> break;<br /> }<br /> }<br /><br /> System.out.println("A: " + count[0]);<br /> System.out.println("T: " + count[1]);<br /> System.out.println("C: " + count[2]);<br /> System.out.println("G: " + count[3]);<br /> }<br />}<br />```<br />2. Object-Oriented Approach:<br />```java<br />public class DNAAnalyser {<br /> private String sequence;<br /><br /> public DNAAnalyser(String sequence) {<br /> this.sequence = sequence;<br /> }<br /><br /> public int[] countNucleotides() {<br /> int[] count = new int[4];<br /> for (int i = 0; i < sequence.length(); i++) {<br /> char nucleotide = sequence.charAt(i);<br /> switch (nucleotide) {<br /> case 'A':<br /> count[0]++;<br /> break;<br /> case 'T':<br /> count[1]++;<br /> break;<br /> case 'C':<br /> count[2]++;<br /> break;<br /> case 'G':<br /> count[3]++;<br /> break;<br /> }<br /> }<br /> return count;<br /> }<br /><br /> public static void main(String[] args) {<br /> String sequence = "ATCGATCGATCG";<br /> DNAAnalyser dna = new DNAAnalyser(sequence);<br /> int[] count = dna.countNucleotides();<br /> System.out.println("A: " + count[0]);<br /> System.out.println("T: " + count[1]);<br /> System.out.println("C: " + count[2]);<br /> System.out.println("G: " + count[3]);<br /> }<br />}<br />```<br />3. Functional Approach:<br />```java<br />import java.util.Arrays;<br />import java.util.Map;<br />import java.util.stream.Collectors;<br /><br />public class DNASequenceAnalysis {<br /> public static void main(String[] args) {<br /> String sequence = "ATCGATCGATCG";<br /> Map<Character, Long> count = sequence.chars()<br /> .collect(Collectors.groupingBy(nucleotide -> nucleotide, Collectors.counting()));<br /><br /> System.out.println("A: " + count.get('A'));<br /> System.out.println("T: " + count.get('T'));<br /> System.out.println("C: " + count.get('C'));<br /> System.out.println("G: " + count.get('G'));<br /> }<br />}<br />```<br />All three approaches will produce the same output for the given input sequence "ATCGATCGATCG":<br />```yaml<br />A: 3<br />T: 3<br />C: 3<br />G: 3<br />```
Derecelendirmek için tıklayın: