Soru
![Part 3 (40pts):
Write a Python function countTypes() that takes a list of mixed data types. The function should count
the number of numbers (integers and floats) and strings in the list and return the counts as a list in the
format [count of __ numbers, count, of strings)
Write Python statements that creates a list containing various data types, call the above function and
print the result.
In your program, you can use the list in the sample run below or create your own list.
Part 3:
The lis t: [1, "hello", 3 .14, True, "world", 42 , [1, 2], "Python", 7.2]](https://static.questionai.com.tr/resource%2Fqaiseoimg%2F202411%2Fpart-3-40ptswrite-python-function-counttypes-takes-list-thTLKISYV80l.jpg?x-oss-process=image/resize,w_600,h_600/quality,q_50/format,webp)
Part 3 (40pts): Write a Python function countTypes() that takes a list of mixed data types. The function should count the number of numbers (integers and floats) and strings in the list and return the counts as a list in the format [count of __ numbers, count, of strings) Write Python statements that creates a list containing various data types, call the above function and print the result. In your program, you can use the list in the sample run below or create your own list. Part 3: The lis t: [1, "hello", 3 .14, True, "world", 42 , [1, 2], "Python", 7.2]
Çözüm
4.5164 Voting
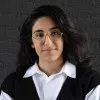
Pınar
Uzman · 3 yıl öğretmeniUzman doğrulaması
Cevap
Here's a Python function that counts the number of numbers and strings in a given list:<br />```python<br />def countTypes(lst):<br /> count = [0, 0]<br /> for item in lst:<br /> if isinstance(item, (int, float)):<br /> count[0] += 1<br /> elif isinstance(item, str):<br /> count[1] += 1<br /> return count<br />```<br />This function takes a list of mixed data types as input and returns a list containing the count of numbers and strings in the format `[count of numbers, count of strings]`.<br /><br />Here's how you can use this function to count the number of numbers and strings in the given list:<br />```python<br /># create a list containing various data types<br />myList = [1, "hello", 3.14, True, "world", 42, [1, 2], "Python", 7.2]<br /><br /># call the countTypes() function and print the result<br />print(countTypes(myList))<br />```<br />When you run this code, it will output the following:<br />```<br />[9, 3]<br />```<br />This means that there are 9 numbers and 3 strings in the given list.
Derecelendirmek için tıklayın: