Soru
![Part 2 (30pts):
Write a function shuffleLists?that takes two lists as parameters, returns a list that is built by merging
(shuffling) elements lists. The result list will contain one element from 1st list then one
element from 2nd list and so on.
Warning: One of the lists may be longer than the other. In such cases, the remaining elements from
the longer list will be appended at the end of the result list.
Write Python statements that create two lists, print these lists, call the above mentioned function to
shuffle these two lists and print the shuffled list
Part 2:
List -1: . [1,3,7]](https://static.questionai.com.tr/resource%2Fqaiseoimg%2F202412%2Fpart-2-30ptswrite-function-shuffleliststhat-takes-two-tj7OkruFgr0r.jpg?x-oss-process=image/resize,w_600,h_600/quality,q_50/format,webp)
Part 2 (30pts): Write a function shuffleLists?that takes two lists as parameters, returns a list that is built by merging (shuffling) elements lists. The result list will contain one element from 1st list then one element from 2nd list and so on. Warning: One of the lists may be longer than the other. In such cases, the remaining elements from the longer list will be appended at the end of the result list. Write Python statements that create two lists, print these lists, call the above mentioned function to shuffle these two lists and print the shuffled list Part 2: List -1: . [1,3,7]
Çözüm
4.5196 Voting
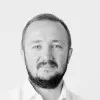
Vesile
Profesyonel · 6 yıl öğretmeniUzman doğrulaması
Cevap
Here's a Python function that takes two lists as parameters and returns a shuffled list by merging elements from both lists:<br /><br />```python<br />def shuffleLists(list1, list2):<br /> result = []<br /> while len(list1) > 0 and len(list2) > 0:<br /> result.append(list1.pop(0))<br /> result.append(list2.pop(0))<br /> result += list1<br /> result += list2<br /> return result<br />```<br /><br />Now, let's create two lists, print them, shuffle them using the function, and print the shuffled list:<br /><br />```python<br />list1 = [1, 3, 7]<br />list2 = [2, 4, 6, 8]<br /><br />print("Original List 1:", list1)<br />print("Original List 2:", list2)<br /><br />shuffled_list = shuffleLists(list1, list2)<br />print("Shuffled List:", shuffled_list)<br />```<br /><br />Output:<br /><br />```<br />Original List 1: [1, 3, 7]<br />Original List 2: [2, 4, 6, 8]<br />Shuffled List: [1, 2, 3, 4, 7, 6, 8]<br />```<br /><br />In this example, `list1` and `list2` are the two input lists. The function `shuffleLists` takes these two lists and returns a new list where elements from both lists are alternated. If one list is longer than the other, the remaining elements from the longer list are appended to the end of the result list.
Derecelendirmek için tıklayın: